Junior Block Documentation
version 2.2.2 (Changelog)
Flight Commands
take off
Block
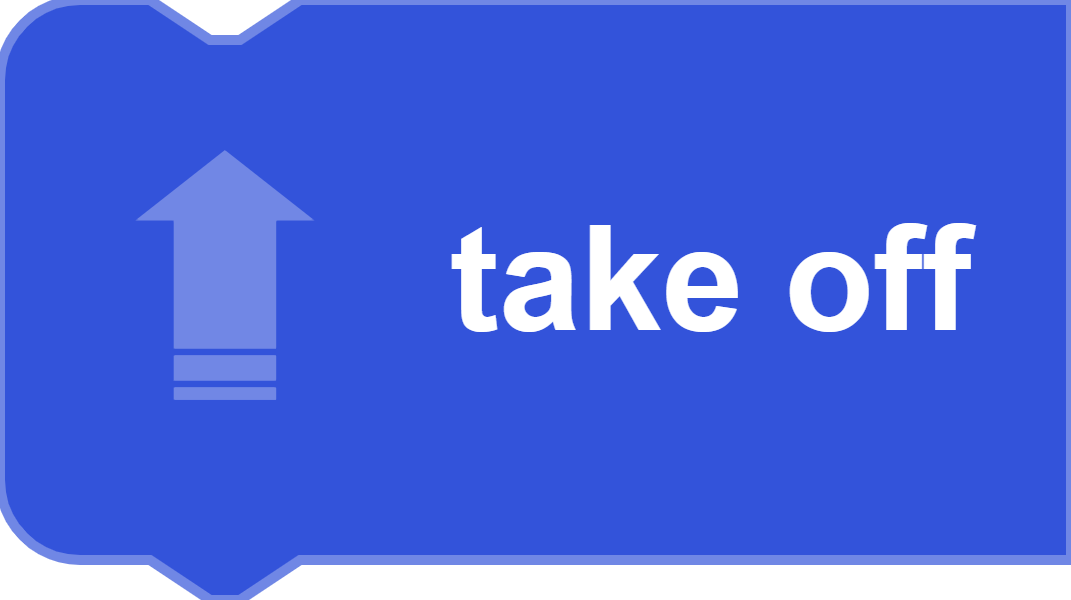
Description
This functions makes the drone take off. CoDrone EDU takes off at an average height of 80 centimeters off the ground. A takeoff block must be used before any other flight command or flight movement. NOTE: The takeoff height cannot be modified.
Parameters
None
Returns
None
Example
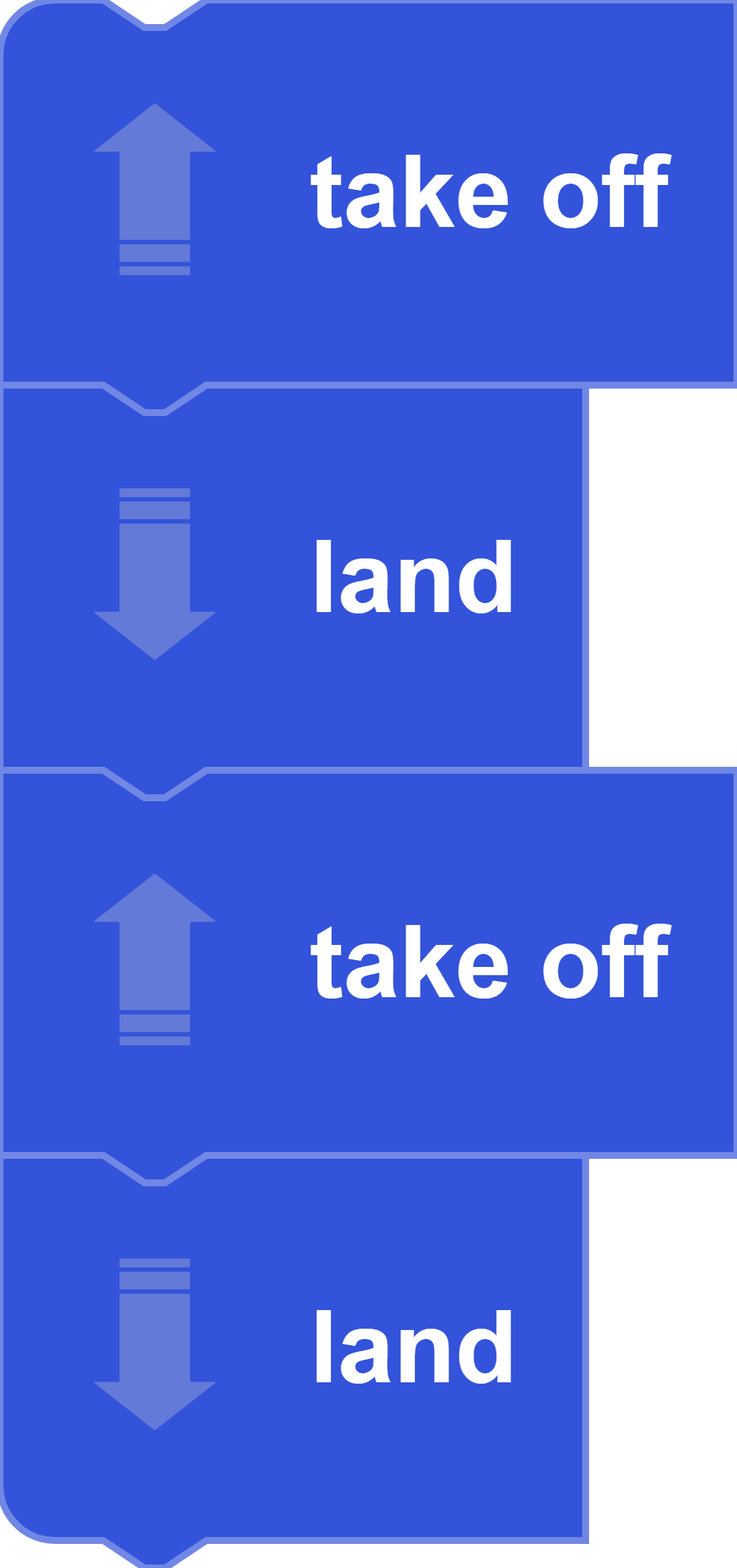
land
Block
Description
This function makes the drone land by throttling down safely.
Parameters
None
Returns
None